Using blender and alternativa3d
I’m not a 3d modeller but with the advancements in flash there are alot of options to create 3d environments on the web and this is something i’ve been itching to do for a while. One of the best technologies to do this in my opinion is alternativa3d. Its alot smoother and seems to run alot better than the others i’ve come across.
If you want to follow the steps i took then you will need to visit http://blender.org and download blender and also visit http://alternativaplatform.com/en/ to download the 3d libraries needed.
Create a 3d model in blender i decided to make the cube with a little roof and floor. I baked the textures and saved it all and exported a .3ds file and images which we will need later. If you aren’t comfortable using blender then you can use the files from my download to continue.
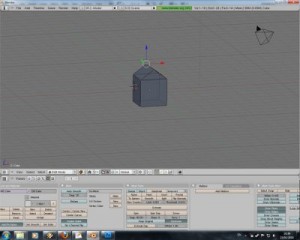
Now to get this working in alternativa3d, we want to convert the .3ds file we made into alternativa3d actionscript classes. You can do this by creating a new flash file.
Open Flash and create a new document it doesn’t matter what dimensions or fps. Create a new keyframe and put the following actionscript in.
import alternativa.utils.*;
import alternativa.engine3d.core.*;
import alternativa.engine3d.loaders.*;
import alternativa.engine3d.materials.TextureMaterialPrecision;
var loader:Loader3DS = new Loader3DS();
loader.smooth = true;
loader.precision = TextureMaterialPrecision.HIGH;
loader.load("house.3ds");
loader.addEventListener(Event.COMPLETE, onLoadingComplete);
function onLoadingComplete(e:Event):void {
for (var o:* in loader.content.children) {
var object:Object3D = o;
trace( MeshUtils.generateClass(Mesh(object), "house") );
}
}
This will essentially open the .3ds file you tell it and then generate an actionscript class using the alternativa meshutility. This class will be output using trace so check your output window for the results and copy and paste it into new .as files. You may need to make some slight changes, as i had 2 meshes it created 2 classes but they both had the same name house so i had to change this slightly to reflect what they are.
Ok so now we have everything we need we can load it all up. So create a new flash document and give it a document class. This will be the code to load the model and create the environment so you can move around etc.
This is the code i have used
package {
//import alternativa libraries needed
import alternativa.types.*;
import alternativa.utils.*;
import alternativa.engine3d.core.*;
import alternativa.engine3d.display.View;
import alternativa.engine3d.controllers.ObjectController;
import alternativa.engine3d.controllers.WalkController;
//import our model classes (they were put in a folder called models)
import models.*;
//import basic flash libraries
import flash.display.*;
import flash.events.*;
public class house extends Sprite {
//these are the variables needed to create the 3d area in flash
private var scene:Scene3D = new Scene3D();
private var sceneRoot:Object3D = scene.root = new Object3D("root");
private var camera:Camera3D = new Camera3D("camera");
private var view:View = new View(camera);
private var controller:WalkController;
//our 3d model classes
private var gnd:Ground = new Ground();
private var hse:House = new House();
public function house():void {
addEventListener(Event.ADDED_TO_STAGE, onAddedToStage);
}
private function onAddedToStage(e:Event):void {
removeEventListener(Event.ADDED_TO_STAGE, onAddedToStage);
stage.align = StageAlign.TOP_LEFT;
stage.scaleMode = StageScaleMode.NO_SCALE;
initScene();
initUI();
addEventListener(Event.ENTER_FRAME, onEnterFrame);
stage.addEventListener(Event.RESIZE, onResize);
onResize();
}
private function initScene():void {
//set the field of view
camera.fov = MathUtils.DEG1*100;
//add the camera and our 2 models to the scene
sceneRoot.addChild(camera);
sceneRoot.addChild(gnd);
sceneRoot.addChild(hse);
//after playing around i decided the models were too small
//so i increased the size here
gnd.scaleX = 5;
gnd.scaleY = 5;
gnd.scaleZ = 5;
hse.scaleX = 5;
hse.scaleY = 5;
hse.scaleZ = 5;
//create our walk controller for movement
controller = new WalkController(stage);
//set the keys
controller.setDefaultBindings();
controller.bindKey(KeyboardUtils.UP, ObjectController.ACTION_FORWARD);
controller.bindKey(KeyboardUtils.DOWN, ObjectController.ACTION_BACK);
controller.bindKey(KeyboardUtils.LEFT, ObjectController.ACTION_LEFT);
controller.bindKey(KeyboardUtils.RIGHT, ObjectController.ACTION_RIGHT);
//controller settings
controller.object = camera;
controller.checkCollisions = true;
controller.speed = 50;
controller.jumpSpeed = 120;
controller.gravity = 700;
controller.objectZPosition = 0.95;
controller.maxGroundAngle = MathUtils.toRadian(45);
controller.speedMultiplier = 2.5;
controller.coords = new Point3D(20, -20, 5);
controller.lookAt(new Point3D(-206.818, 147.854, 52));
controller.collider.offsetThreshold = 0.0001;
controller.collider.radiusX = 1;
controller.collider.radiusY = 1;
controller.collider.radiusZ = 5;
//calculate the scene
scene.calculate();
}
private function initUI():void {
addChild(view);
view.interactive = true;
FPS.init(this);
}
private function onEnterFrame(e:Event = null):void {
controller.processInput();
scene.calculate();
}
private function onResize(e:Event = null):void {
view.width = stage.stageWidth;
view.height = stage.stageHeight;
onEnterFrame();
}
}
}
Now there is one last thing we need to do for this to work. We need to add the textures to the models, so import your images into the flash document and give them classes. I called mine floort and houset which have the base class of flash.display.BitmapData.
Ok so now we need to alter our model classes. The code that is output for them from the meshutility is actually for flex as it uses the embed method for the images so we will change this slightly.
So for the house model for example it had the following:
[Embed(source="house.jpg")] private static const bmpHouse:Class;
private static const house:Texture = new Texture(new bmpHouse().bitmapData, "house.jpg");
We need to change this to:
private static var hbmd:houset = new houset(0,0);
private static const house:Texture = new Texture(hbmd, "house.jpg");
Again we need to do this to all the classes we have. Once that is done we should be good to go. Try running the flash file and see if it works. If you did everything correctly you should see the 3d model in your flash document and you can move around.
If you are having problems seeing your model you may need to play around with the controller settings specifically the coords and lookat values:
controller.coords = new Point3D(20, -20, 5);
controller.lookAt(new Point3D(-206.818, 147.854, 52));
Comments
Comments are currently closed